Trading Card FX Shader
This card shader was made for Harry Alisavakis’s technical art challenge
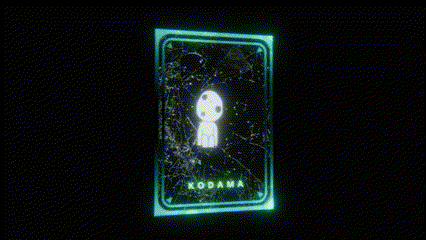
In turn based games trading cards are very popular. Different techniques in shader can be used to make it look interesting to the players. In this asset, I tried to create a magic card that has a fake depth. This parallax effect was achieved by offsetting the view direction vector.
. . //Transform the view direction from world space to tangent space float3 worldVertexPos = mul(unity_ObjectToWorld, v.vertex).xyz; float3 worldViewDir = worldVertexPos - _WorldSpaceCameraPos; //To convert from world space to tangent space we need the following //https://docs.unity3d.com/Manual/SL-VertexFragmentShaderExamples.html float3 worldNormal = UnityObjectToWorldNormal(v.normal); float3 worldTangent = UnityObjectToWorldDir(v.tangent.xyz); float3 worldBitangent = cross(worldNormal, worldTangent) * v.tangent.w * unity_WorldTransformParams.w; //Use dot products instead of building the matrix o.tangentViewDir = float3( dot(worldViewDir, worldTangent), dot(worldViewDir, worldBitangent), dot(worldViewDir, worldNormal) ); . .
I used different noise patterns for the foil reflection and broken glass textures.
. . float pattern = (sin((i.viewDir.z ) * _GradientTile * UNITY_TWO_PI) * 0.5 + 0.5) * fresnel ; fixed4 gradient = tex2D(_GradientTex,pattern); float4 texCenter = (noiseLayer1 + noiseLayer2) * _ColorInside * dotProduct * (1-mask); float4 texBorder = (gradient + _Metal ) * _Light * _Color * (mask ) * fresnel ; float4 col = texCenter + texBorder ; . .